⬅️ Variable practice ➡️
Variables and Data Types in Python
Welcome to the exciting world of variables! In Python, variables are like magical containers that can hold different types of information. Let's explore some of the most common ones.
Data Types: Different Flavours of Information
Just like ice cream comes in different flavours, data in Python comes in different types:
Data types | Meaning | Example |
---|---|---|
Strings | Alphanumeric characters - text wrapped in quotes | "Hello, Python!" |
Integers | Whole numbers | 42 or -10 |
Floats | Numbers with decimal points | 3.14 or -0.5 |
Input: Getting Information from Users
Remember the input()
function? It's super helpful,
but it has a secret: it always gives us a string, even if the
user types a number!
age = input("How old are you? ")
If the user enters 12, the variable age
will be the
string "12"
, not the integer 12
.
Casting: Changing Data Types
Sometimes we need to change the type of our data. We can do this with casting:
age = int(input("How old are you? "))
Now if the user enters 12, the variable age
will be
the integer 12
, which we can use in maths!
Mathematical Operators: Doing Maths with Python
Python is great at maths! Here are some operators you can use:
Operator | Function |
---|---|
+ |
addition |
- |
subtraction |
* |
multiplication |
/ |
division |
Updating Variables: Changing Values
Variables are not only for storing values - we can also change them as our program runs. This is super useful for keeping track of things that change, like scores in a game!
Here's how we can update a variable:
num = 4
print("num starts as:", num)
num = num + 2
print("After adding 2, num is now:", num)
In this example, num
starts as 4. Then we add 2 to
it and store the result back in num
. So
num
becomes 6!
We can do this with any mathematical operation. Here are a few more examples:
num = num * 3 # Multiplies num by 3
num = num - 1 # Subtracts 1 from num
num = num / 2 # Divides num by 2
This is really powerful because it lets our variables change and grow as our program runs. Try using this in your next program!
Putting It All Together
Let's use everything we've learned to make a cool program:
days = int(input("Give me a number of days: "))
hours = days * 24
print("There are", hours, "hours in", days, "days")
This program asks for a number of days, casts the response to an integer, calculates the number of hours, and then prints the result.
Now it's your turn! Can you make a program that asks for someone's age and then calculates how many months they've been alive? (Hint: multiply their age by 12!) Press inspire me! for more ideas of what to code.
Write a program to...
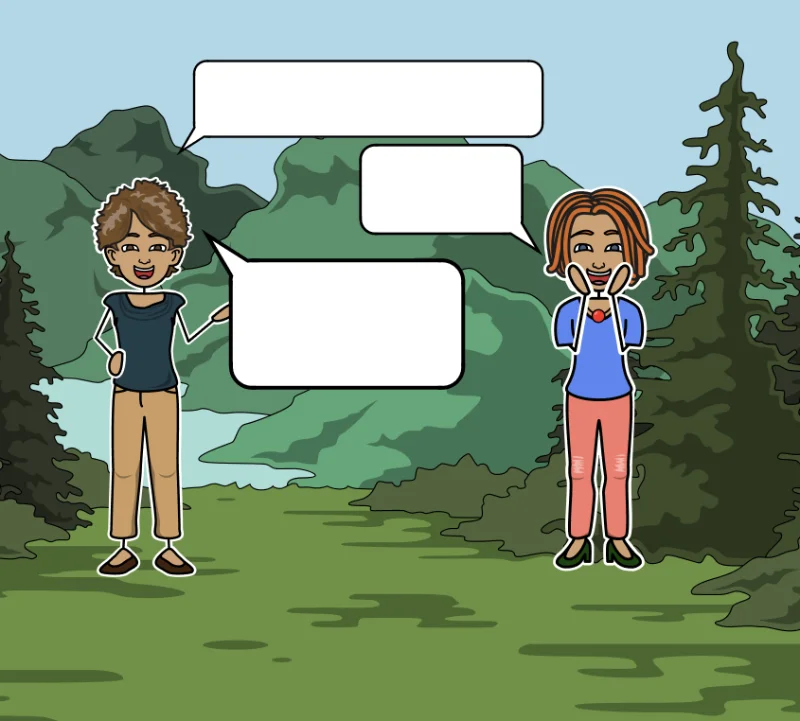
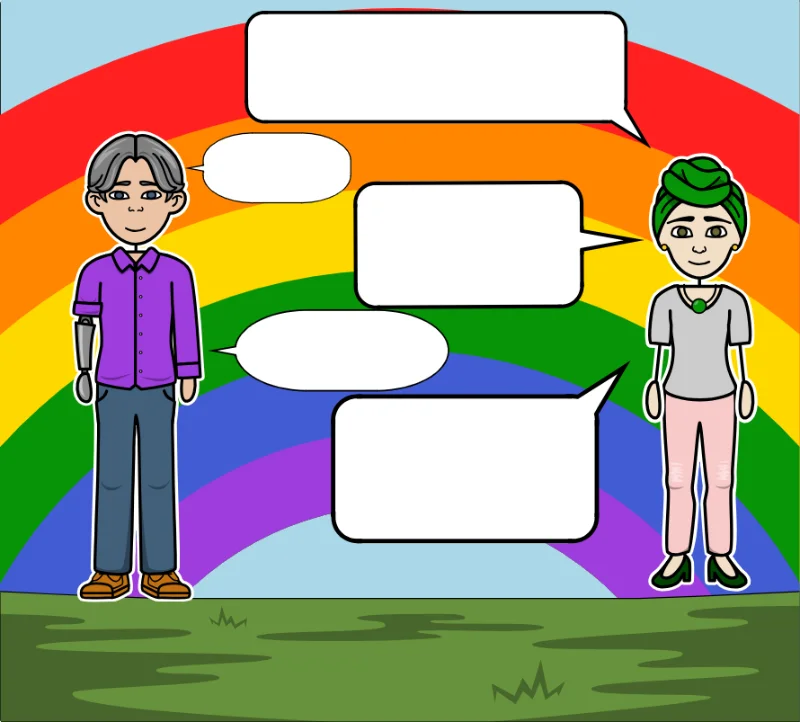