⬅️ Selection practice with if/else ➡️
Selection in Python
Welcome to the world of decision-making in programming! Today, we're going to learn about selection, which is like teaching your computer to make choices. It's a bit like being the director of your own interactive movie!
The Three Musketeers of Programming
In programming, we have three main ways to control how our code runs. These are called programming constructs:
Construct | Meaning |
---|---|
sequence | This is like following a recipe step by step and in order. |
selection | This is about making choices, today's task, uses if, elif, else. |
iteration | This is about repeating things, but that's a story for another day. |
Today, we're focusing on selection, which is all about making decisions in our code.
If-Else: Teaching Your Computer to Make Choices
In Python, we use 'if' and 'else' statements to make decisions. It's like asking a question and doing different things based on the answer.
if it_is_raining:
print("Take an umbrella")
else:
print("Enjoy the sunshine")
In this example, if it's raining, the program tells you to take an umbrella. Otherwise (else), it tells you to enjoy the sunshine.
The Tale of Two Equals: = vs ==
In Python, we use equals signs in two different ways:
Operator | Meaning |
---|---|
= |
This is for assigning values to variables. It's like putting something in a box. |
== |
This is for comparing values. It's like asking "Are these the same?" |
favourite_colour = "blue" # Assigning a value
if favourite_colour == "blue": # Comparing values
print("Great choice!")
The Importance of Indentation
In Python, indentation (the space at the beginning of a line) is super important! It tells Python which lines of code belong to an if statement.
After an if or else statement, you need to:
- Put a colon (:) at the end of the line
- Indent the next line (usually by pressing the Tab key once)
if score > 10:
print("You win!") # This line is
indented
print("Well done!") # This line is
also indented
print("Game over") # This line is not indented, so it's not
part of the if
Putting It All Together
Let's use everything we've learned to make a fun program:
correct_answer = "spongebob squarepants"
best_show = input("What is the best TV show? ")
if best_show == correct_answer:
print("Absolutely!", correct_answer,
"is glorious")
else:
print(best_show, "?!?! No way - it
is", correct_answer)
This program asks for your favourite TV show. If you say "spongebob squarepants", it agrees with you. If you say anything else, it tells you that Spongebob is actually the best!
Now it's your turn! Can you make a program that asks for a password and only lets the user in if they enter the correct one? Press inspire me for more ideas of what to code.
Write a program to...
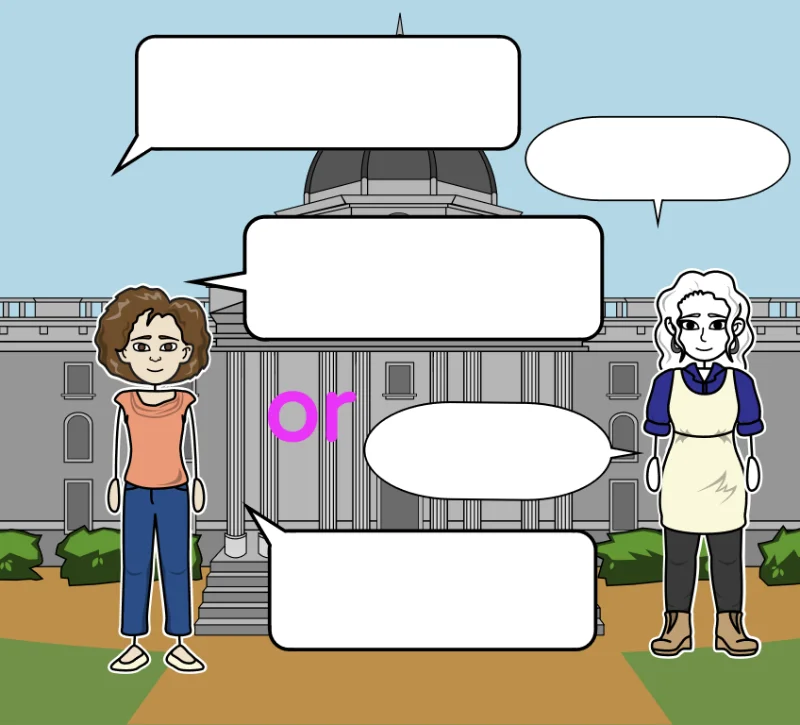
else: